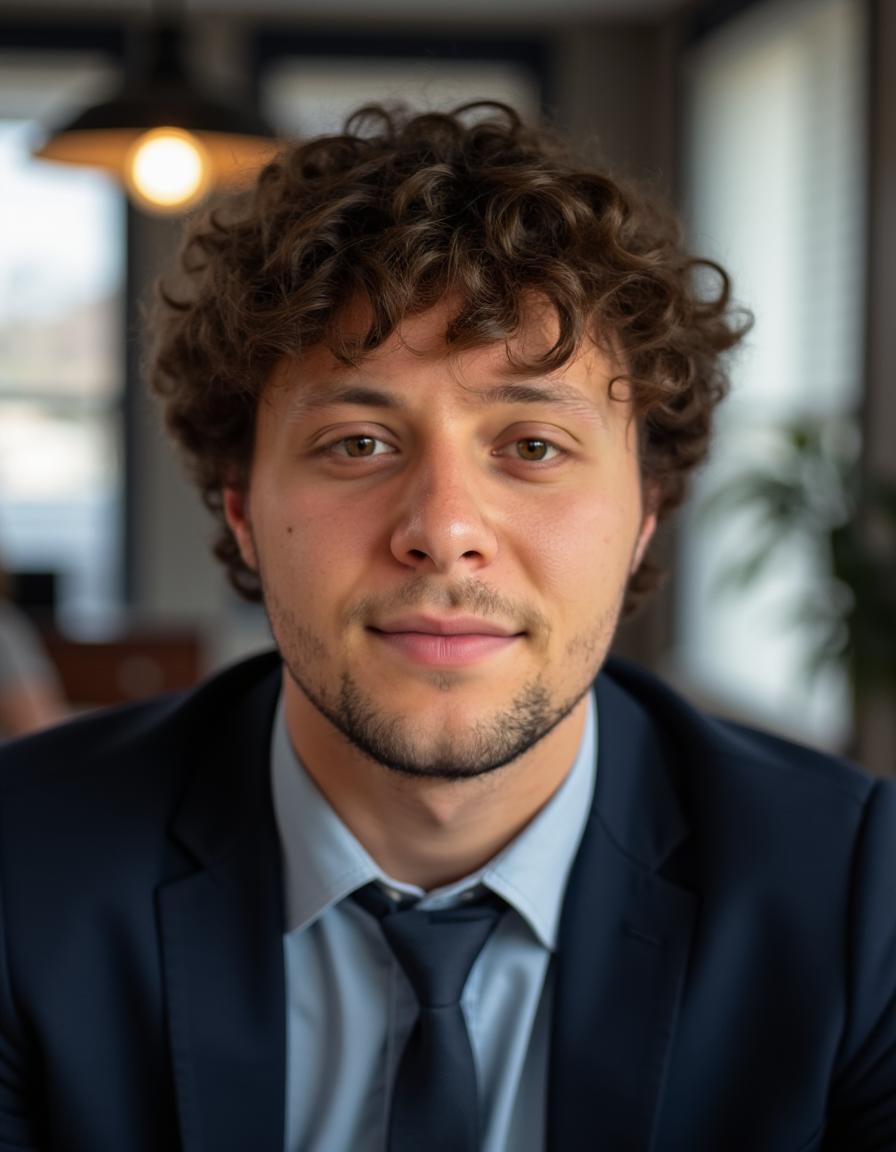
Mastering Vue.js: A Guide to Building Interactive Web Apps
Vue.js is a **progressive JavaScript framework** for building dynamic and reactive user interfaces. It is lightweight, flexible, and easy to integrate into existing projects.
Why Choose Vue.js?
Vue.js offers:
- Declarative Rendering - Uses template syntax to bind data.
- Reactivity System - Automatically updates the DOM.
- Component-Based Architecture - Reusable UI elements.
- Directives - Special attributes like `v-if`, `v-for`, `v-model`.
- Vue Router & Vuex - Powerful state management and routing.
Setting Up a Vue Project
You can install Vue.js using Vue CLI:
npm install -g @vue/cli
vue create my-vue-app
cd my-vue-app
npm run serve
Vue Components
Vue applications are built using **components**, which encapsulate UI and logic.
Single-File Component Example
<template>
<div>
<h1>Hello, Vue!</h1>
</div>
</template>
<script>
export default {
name: 'HelloWorld'
}
</script>
<style scoped>
h1 { color: #42b983; }
</style>
Reactivity & Data Binding
Vue.js **reactively updates the DOM** when data changes.
Reactive Data Example
<template>
<div>
<p>Message: {{ message }}</p>
<button @click="updateMessage">Update Message</button>
</div>
</template>
<script>
export default {
data() {
return {
message: "Hello, Vue!"
};
},
methods: {
updateMessage() {
this.message = "Vue is awesome!";
}
}
}
</script>
Vue Directives
Vue uses **directives** to extend HTML with special attributes.
Common Directives
- `v-if` - Conditional rendering.
- `v-for` - Loop through arrays.
- `v-model` - Two-way data binding.
- `v-bind` - Bind attributes dynamically.
Example: v-if & v-for
<template>
<div>
<p v-if="showMessage">This message is visible!</p>
<ul>
<li v-for="item in items" :key="item.id"> {{ item.name }} </li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
showMessage: true,
items: [
{ id: 1, name: "Item 1" },
{ id: 2, name: "Item 2" },
{ id: 3, name: "Item 3" }
]
};
}
}
</script>
Vue Router & State Management
- Vue Router - Manage page navigation.
- Vuex - Centralized state management.
Vue Router Example
npm install vue-router
🚀 Conclusion
Vue.js is an excellent **lightweight framework** for building **scalable and reactive** applications.