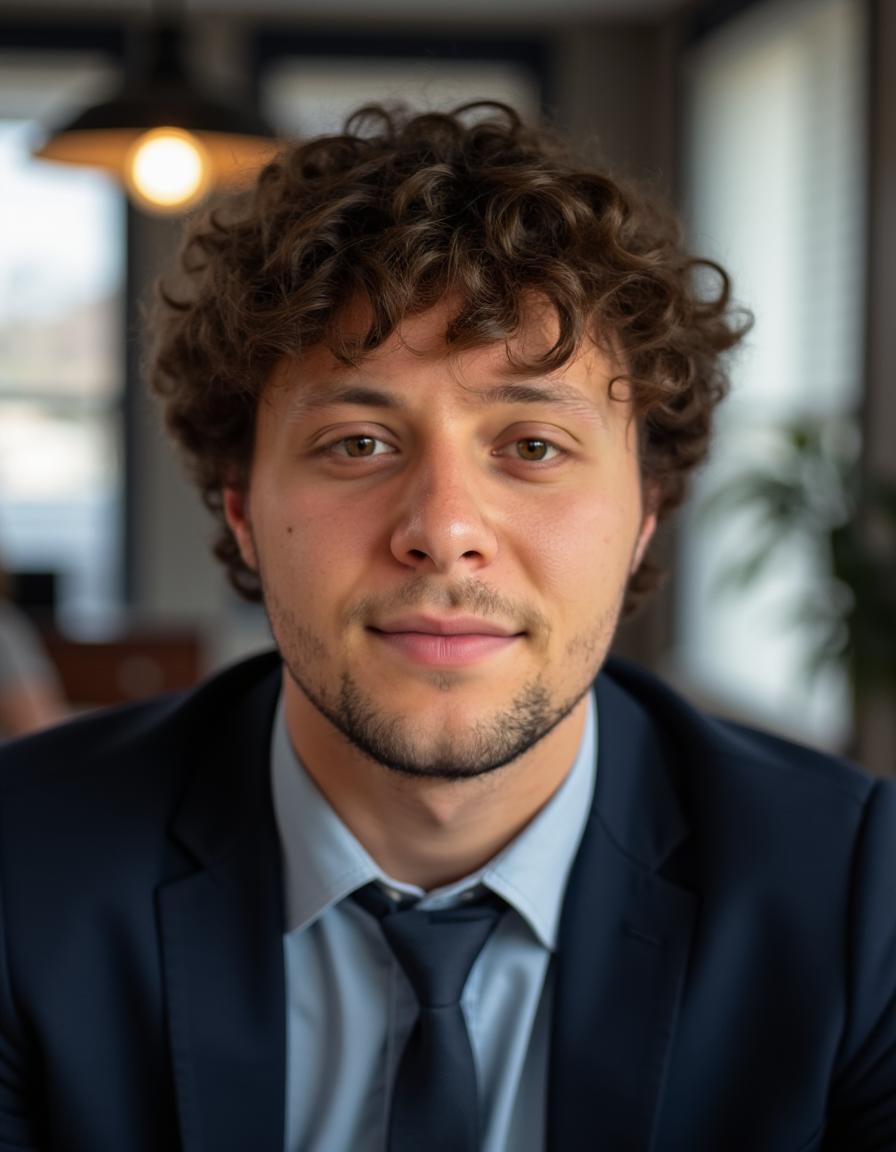
Mastering Unity: The Ultimate Game Development Engine
Unity is a powerful, **cross-platform game engine** widely used for developing **2D, 3D, AR, and VR games**. With its **C# scripting**, robust physics engine, and extensive asset store, Unity makes game development accessible to beginners and professionals alike.
Why Use Unity?
Unity offers several advantages for game developers:
- Cross-Platform - Build games for PC, mobile, consoles, and VR.
- C# Scripting - Powerful and beginner-friendly programming.
- Physics & AI - Advanced physics engine and AI behavior tools.
- Asset Store - Access thousands of 3D models, scripts, and tools.
- Built-in Monetization - Unity Ads, In-App Purchases, and analytics.
Installing Unity
To install Unity, download **Unity Hub** from the official website:
https://unity.com/download
Creating a New Unity Project
Once Unity Hub is installed, create a new project:
1. Open Unity Hub
2. Click "New Project"
3. Select 2D, 3D, or other templates
4. Name your project and select a save location
5. Click "Create"
Writing Your First C# Script
In Unity, scripts are written in **C#** and attached to GameObjects.
using UnityEngine;
public class HelloWorld : MonoBehaviour
{
void Start()
{
Debug.Log("Hello, Unity!");
}
}
Attach this script to a GameObject in the Unity Editor and press **Play** to see the message in the **Console**.
Handling Player Movement
Let's add basic **player movement** using Unity's Input System:
using UnityEngine;
public class PlayerMovement : MonoBehaviour
{
public float speed = 5f;
void Update()
{
float moveX = Input.GetAxis("Horizontal");
float moveY = Input.GetAxis("Vertical");
transform.Translate(new Vector3(moveX, moveY, 0) * speed * Time.deltaTime);
}
}
Attach this script to a **2D sprite** or **3D character** and use the **WASD** or **Arrow Keys** to move.
Adding a Jump Mechanic
A simple **jumping mechanic** using Unity's physics engine:
using UnityEngine;
public class PlayerJump : MonoBehaviour
{
public float jumpForce = 7f;
private Rigidbody2D rb;
void Start()
{
rb = GetComponent();
}
void Update()
{
if (Input.GetKeyDown(KeyCode.Space))
{
rb.velocity = new Vector2(rb.velocity.x, jumpForce);
}
}
}
This script allows the player to jump when pressing the **Spacebar**.
Building Your Game
Once your game is ready, export it for different platforms:
1. Go to "File" → "Build Settings"
2. Select a platform (Windows, Mac, Linux, Android, iOS, etc.)
3. Click "Switch Platform"
4. Adjust settings, then click "Build & Run"
Conclusion
Unity is a **powerful and flexible game engine** that enables you to bring your ideas to life. Whether you're making a 2D platformer, a 3D RPG, or a VR experience, **Unity has the tools to help you succeed**. Start experimenting, and happy coding!