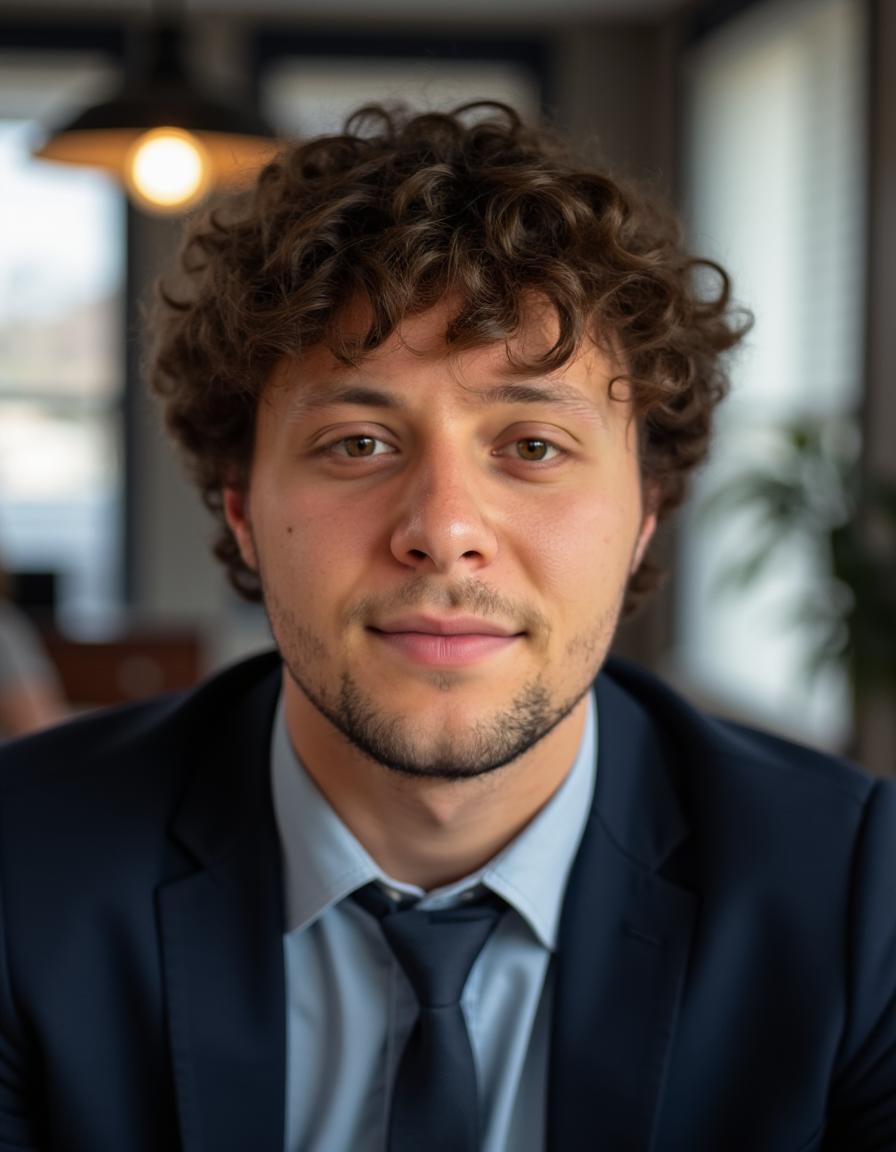
Mastering React: A Guide for Modern Web Development
React is a **declarative, component-based JavaScript library** for building user interfaces. It allows developers to build **fast, scalable, and dynamic** web applications.
Why Choose React?
React simplifies UI development with:
- Component-Based Architecture - Reusable, modular components.
- Virtual DOM - Efficient UI rendering.
- Hooks - Manage state and side effects.
- One-Way Data Flow - Predictable state management.
- JSX - JavaScript syntax for UI structures.
Setting Up a React Project
To create a React app, use the following command:
npx create-react-app my-app
cd my-app
npm start
Understanding Components
React applications are **built using components**. A component can be either a **function-based** or a **class-based** component.
Functional Component Example
import React from 'react';
const Welcome = () => {
return Hello, React!
;
};
export default Welcome;
Class Component Example
import React, { Component } from 'react';
class Welcome extends Component {
render() {
return Hello, React!
;
}
}
export default Welcome;
Managing State with Hooks
React introduced **Hooks** in version 16.8, allowing functional components to **manage state and lifecycle methods**.
Using the `useState` Hook
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
return (
Count: {count}
);
};
export default Counter;
Handling Events in React
React handles **events** similarly to regular JavaScript but follows a **synthetic event system**.
Event Handling Example
import React from 'react';
const ButtonClick = () => {
const handleClick = () => {
alert('Button Clicked!');
};
return ;
};
export default ButtonClick;
Optimizing Performance
React offers several techniques for **performance optimization**:
- React.memo() - Prevents unnecessary re-renders.
- useCallback() - Memoizes functions.
- useMemo() - Optimizes expensive calculations.
- Lazy Loading - Loads components only when needed.
Using `React.memo()`
import React, { memo } from 'react';
const ExpensiveComponent = memo(({ data }) => {
console.log('Rendering Expensive Component');
return {data};
});
export default ExpensiveComponent;
🚀 Conclusion
React is a **powerful, flexible, and modern** library for UI development. Mastering its features will help you **build fast and scalable** web apps.