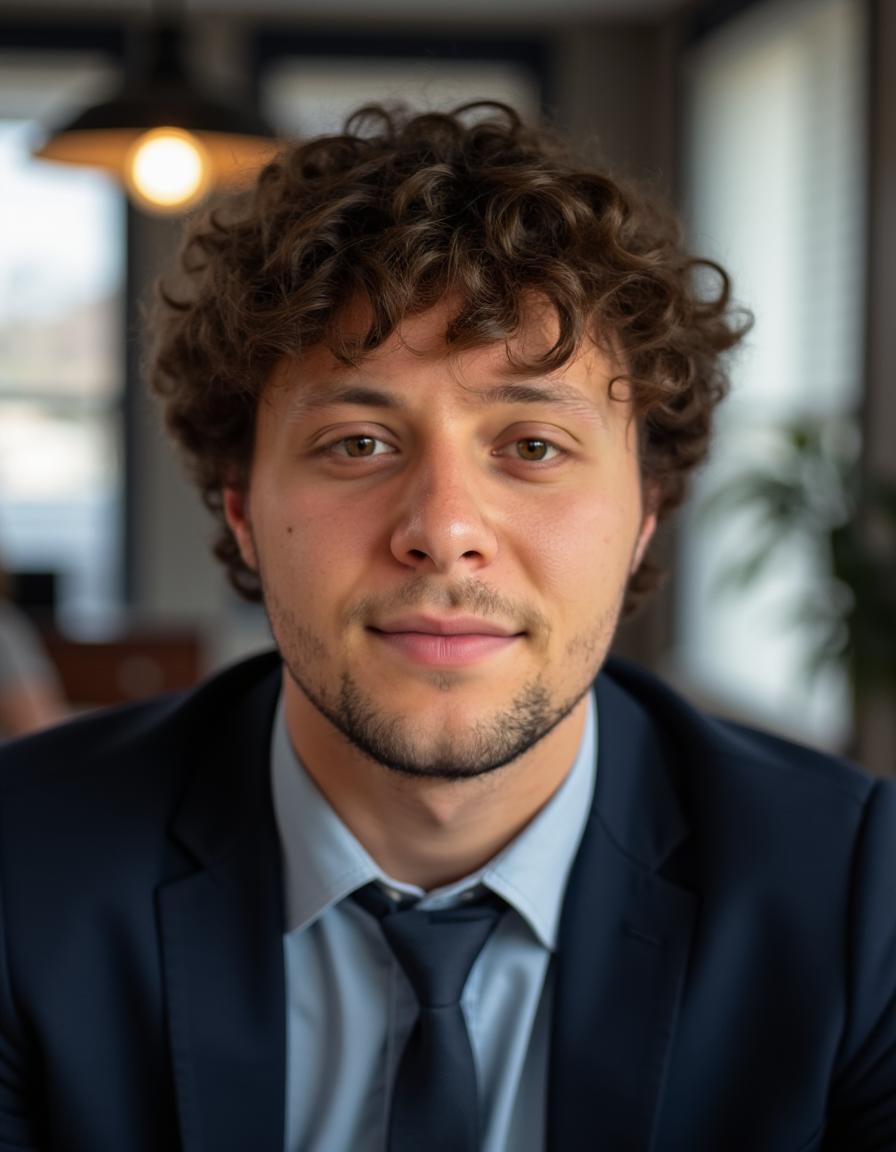
Mastering Python: The Versatile Language for Developers
Python is a **high-level, interpreted** programming language known for its simplicity and versatility. It is widely used in **web development, data science, AI, automation, and more**.
Why Learn Python?
Python has several advantages that make it one of the most popular programming languages:
- Easy to Read & Write - Simple and human-readable syntax.
- Versatile - Used for web development, automation, AI, and more.
- Massive Community - Large support network and extensive libraries.
- Cross-Platform - Runs on Windows, macOS, and Linux.
- Strong Libraries - Pandas, NumPy, TensorFlow, Flask, Django, etc.
Installing Python
You can install Python from the official website:
sudo apt install python3
Verify the installation:
python3 --version
Writing Your First Python Program
Create a **Hello, World!** script:
# Python Hello World
print("Hello, World!")
Run the script:
python3 script.py
Using Python for Web Development
Python is widely used in **backend development** with Flask and Django.
from flask import Flask
app = Flask(__name__)
@app.route("/")
def hello():
return "Hello, Flask!"
if __name__ == "__main__":
app.run(debug=True)
Python for Data Science
Python has powerful libraries for **data analysis and machine learning**:
import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]}
df = pd.DataFrame(data)
print(df)
Python for Automation
Use Python to automate repetitive tasks:
import os
for file in os.listdir("."):
if file.endswith(".txt"):
print("Found text file:", file)
Conclusion
Python is **powerful, versatile, and beginner-friendly**. Whether you're building web applications, automating tasks, analyzing data, or diving into AI, Python is an **essential** tool for modern developers.