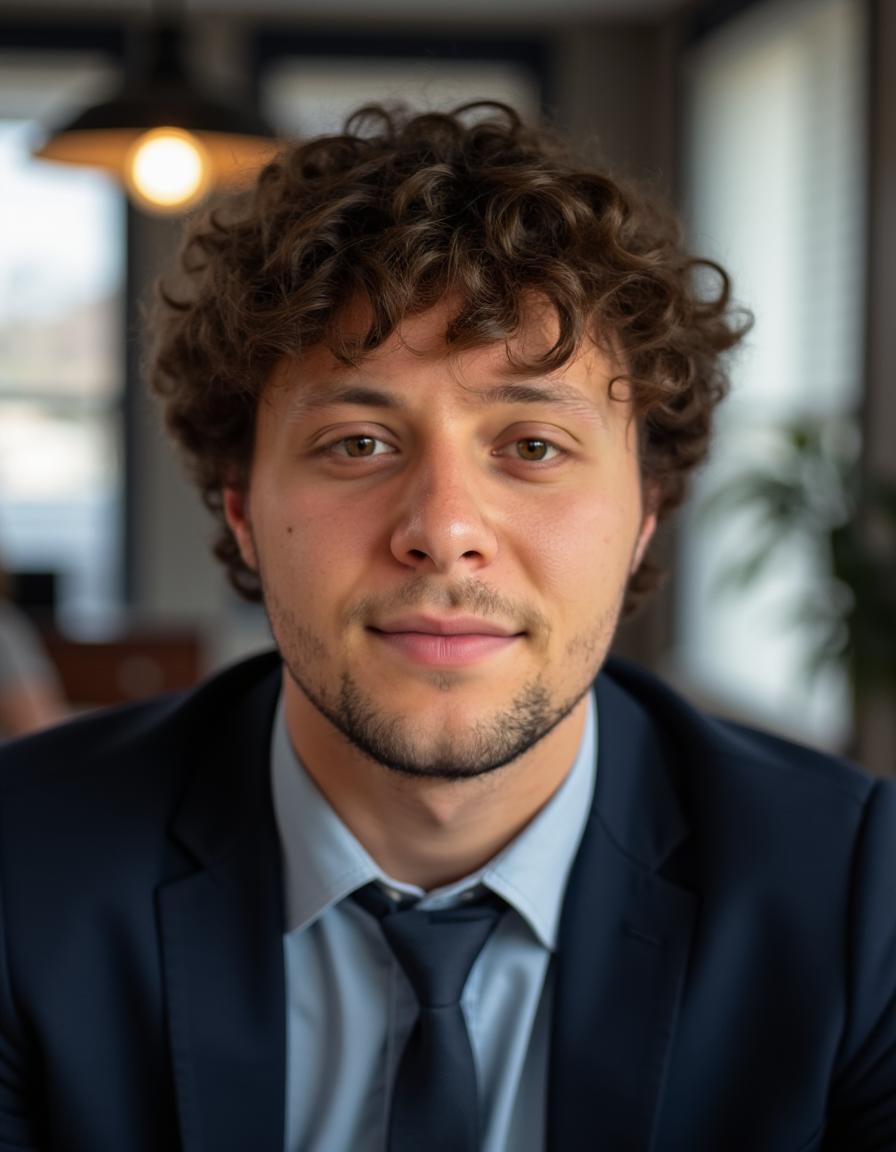
Procedural Generation: Creating Dynamic Worlds in Games
Procedural generation is a powerful technique used in game development to create **randomized and infinite content**. It enables dynamic world-building, random level generation, and unique player experiences by using **algorithms** instead of manually placed elements.
Why Use Procedural Generation?
Procedural generation offers multiple advantages in game development:
- Infinite Worlds - Create endless levels and environments.
- Replayability - Each playthrough offers unique experiences.
- Efficiency - Reduce manual level design effort.
- Performance Optimization - Load chunks dynamically rather than storing everything.
Basic Concepts of Procedural Generation
Procedural generation relies on algorithms to generate content dynamically. Some core concepts include:
1. **Random Noise** - Generates pseudo-random values for landscapes (e.g., Perlin noise, Simplex noise).
2. **Tile-based Generation** - Uses predefined tiles combined randomly to create levels.
3. **Rule-Based Systems** - Applies constraints to ensure generated content follows specific rules.
4. **Procedural Textures** - Generates dynamic textures at runtime for diverse environments.
Generating Terrain with Perlin Noise
Perlin noise is commonly used for terrain generation in Unity.
using UnityEngine;
public class ProceduralTerrain : MonoBehaviour
{
public int width = 256;
public int height = 256;
public float scale = 20f;
void Start()
{
GenerateTerrain();
}
void GenerateTerrain()
{
Terrain terrain = GetComponent();
terrain.terrainData = GenerateTerrainData(terrain.terrainData);
}
TerrainData GenerateTerrainData(TerrainData terrainData)
{
terrainData.heightmapResolution = width + 1;
terrainData.size = new Vector3(width, 50, height);
terrainData.SetHeights(0, 0, GenerateHeights());
return terrainData;
}
float[,] GenerateHeights()
{
float[,] heights = new float[width, height];
for (int x = 0; x < width; x++)
{
for (int y = 0; y < height; y++)
{
heights[x, y] = Mathf.PerlinNoise(x * scale / width, y * scale / height);
}
}
return heights;
}
}
Procedural Dungeon Generation
Randomized dungeon generation is commonly used in **roguelikes** and **RPGs**.
using UnityEngine;
public class DungeonGenerator : MonoBehaviour
{
public int dungeonWidth = 10;
public int dungeonHeight = 10;
public GameObject wallPrefab;
public GameObject floorPrefab;
void Start()
{
GenerateDungeon();
}
void GenerateDungeon()
{
for (int x = 0; x < dungeonWidth; x++)
{
for (int y = 0; y < dungeonHeight; y++)
{
Vector3 position = new Vector3(x, 0, y);
Instantiate(Random.value > 0.8f ? wallPrefab : floorPrefab, position, Quaternion.identity);
}
}
}
}
Tile-based Level Generation
Grid-based procedural generation is useful for **platformers and strategy games**.
using UnityEngine;
public class TileGenerator : MonoBehaviour
{
public GameObject tilePrefab;
public int gridSize = 10;
void Start()
{
GenerateTiles();
}
void GenerateTiles()
{
for (int x = 0; x < gridSize; x++)
{
for (int y = 0; y < gridSize; y++)
{
Vector3 position = new Vector3(x, 0, y);
Instantiate(tilePrefab, position, Quaternion.identity);
}
}
}
}
Conclusion
Procedural generation enables **endless replayability** and **dynamic worlds** in games. Whether it's terrain, dungeons, or grid-based levels, these techniques provide infinite possibilities for **level design, game variety, and content generation**. Start experimenting with different algorithms and create unique game worlds today!