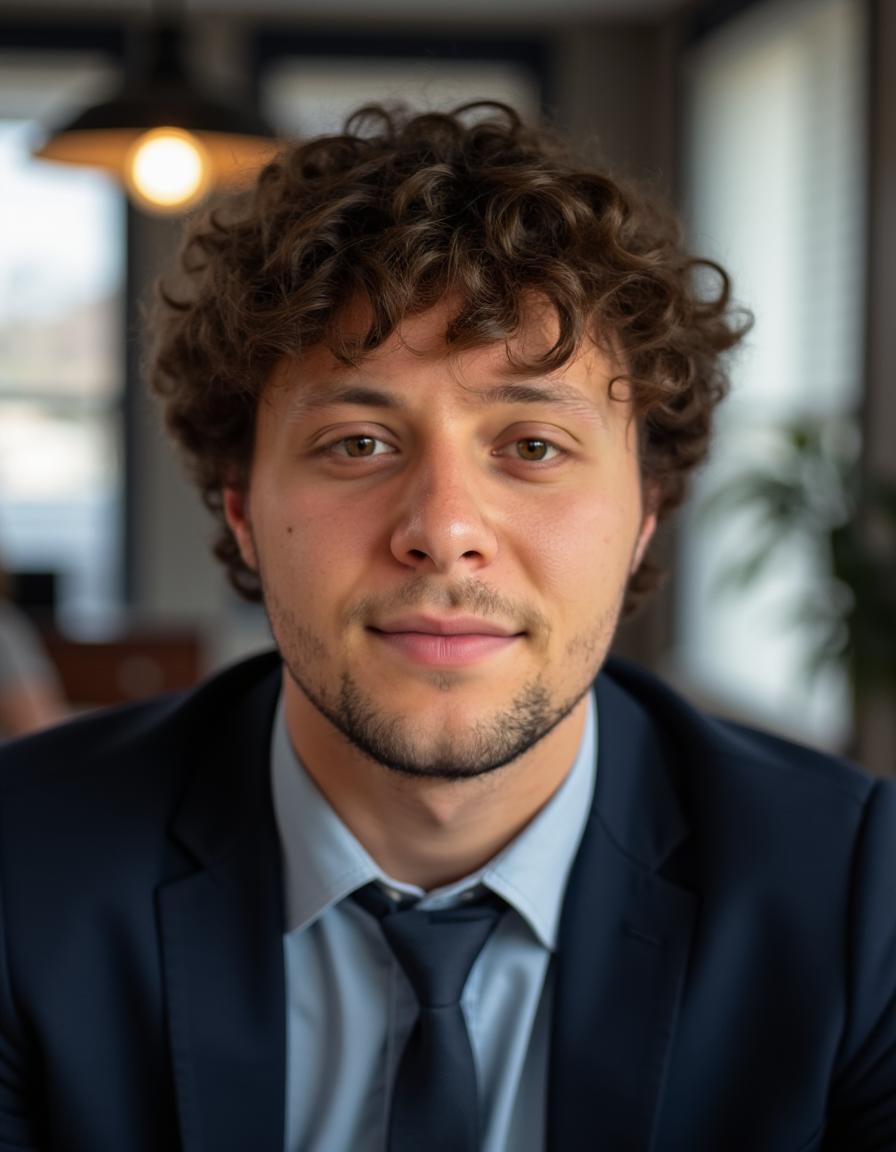
Mastering PHP: A Beginner to Advanced Guide
**PHP** (Hypertext Preprocessor) is a powerful, server-side scripting language designed for web development. It is widely used to build **dynamic websites and APIs** and integrates seamlessly with databases like MySQL.
Why Use PHP?
PHP offers a wide range of benefits:
- Easy to Learn - Simple syntax and fast development.
- Server-Side Execution - Generates dynamic content.
- Database Integration - Works with MySQL, PostgreSQL, and more.
- Framework Support - Laravel, Symfony, and CodeIgniter.
- Cross-Platform - Runs on Windows, Linux, and macOS.
Installing PHP
Download and install PHP from the official website:
sudo apt install php
Check if PHP is installed:
php -v
Writing Your First PHP Script
Create a basic **Hello, World!** script:
<?php
echo "Hello, World!";
?>
Run the script in your terminal:
php script.php
Connecting to a Database
Use PHP to connect to a MySQL database:
<?php
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "my_database";
$conn = new mysqli($servername, $username, $password, $dbname);
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
echo "Connected successfully!";
?>
Using PHP with Laravel
Laravel is a PHP framework that simplifies web development:
composer create-project laravel/laravel my-project
Conclusion
PHP remains one of the **most versatile** and widely used languages for web development. Whether youβre building simple websites or complex applications with Laravel, PHP provides the flexibility and power needed.