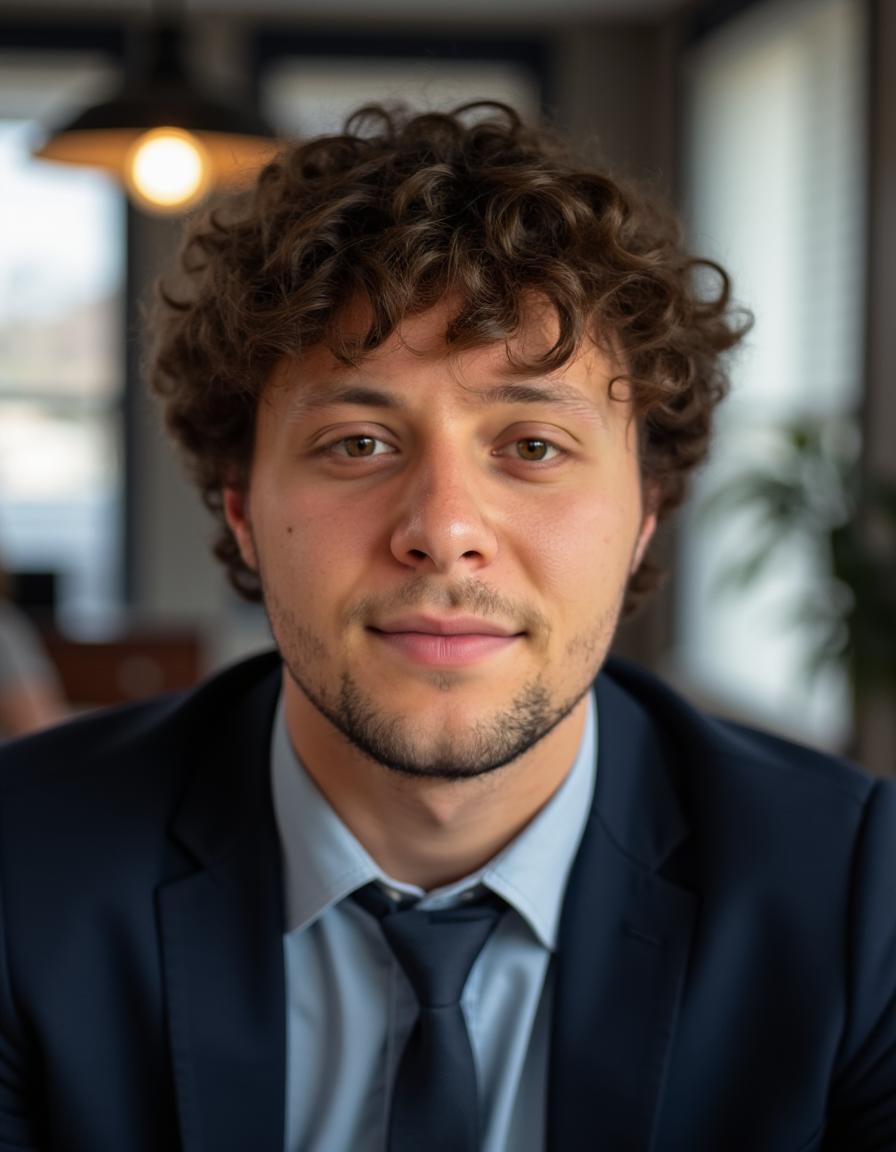
Mastering Node.js: Backend Development with JavaScript
**Node.js** is a powerful **JavaScript runtime** built on Chromeโs V8 engine, enabling **fast, scalable, and efficient server-side applications**. It is widely used for building APIs, microservices, and real-time applications.
Why Use Node.js?
Node.js offers several advantages for backend development:
- Asynchronous & Non-blocking - Handles multiple requests efficiently.
- Fast Performance - Powered by Googleโs V8 engine.
- Single Programming Language - JavaScript for both frontend & backend.
- NPM Ecosystem - Access thousands of open-source packages.
- Scalability - Ideal for microservices and real-time applications.
Installing Node.js
You can download and install Node.js from the official website:
npm install -g node
Check if Node.js is installed:
node -v
Creating a Simple Node.js Server
Create a basic HTTP server using **Node.js**:
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello, Node.js!');
});
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
Run the server with:
node server.js
Using Express.js for Web Applications
Express.js is a **popular web framework** for Node.js.
npm install express
Building an API with Express.js
Hereโs a simple **REST API** using Express.js:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Welcome to my API!');
});
app.listen(3000, () => {
console.log('API running on http://localhost:3000/');
});
Conclusion
Node.js is a **game-changer** for modern web development. With its **asynchronous** nature, rich package ecosystem, and seamless JavaScript integration, it enables powerful and efficient backend solutions.