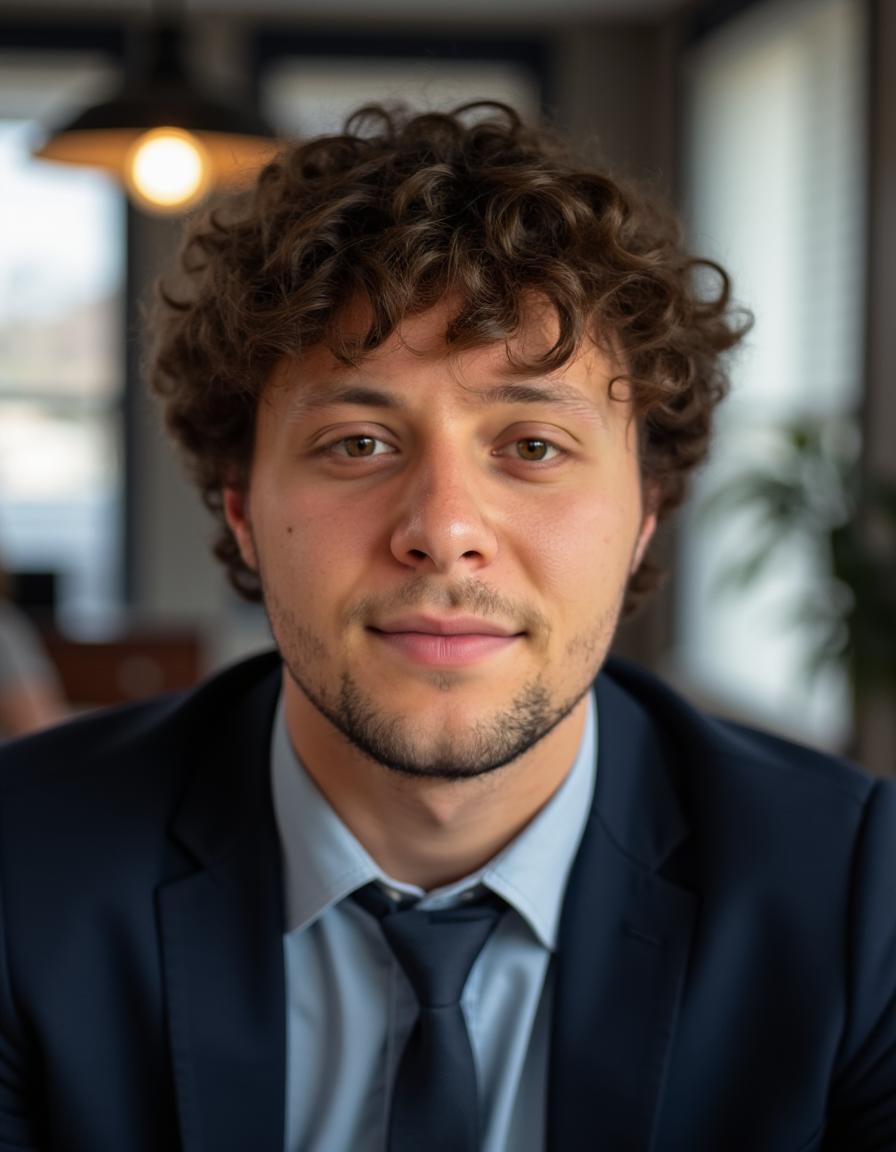
Mastering Multiplayer Networking in Unity
Multiplayer networking allows developers to create interactive online games where players can **connect, interact, and compete**. Unity provides several networking solutions, including **Mirror, FishNet, Photon, and Unity Netcode**. This guide explores the fundamentals of **multiplayer networking** in Unity and how to get started.
Why Use Multiplayer Networking?
Networking is essential for **online gaming**. It allows:
- Real-time Player Interaction - Enable players to interact dynamically.
- Competitive & Cooperative Modes - Build multiplayer combat or co-op experiences.
- Dedicated Server Hosting - Reduce latency and improve stability.
- Cross-Platform Play - Connect players across different devices.
Choosing a Networking Solution
Unity supports multiple **networking frameworks**:
1. **Mirror** - Open-source, optimized for performance, recommended for most multiplayer games.
2. **FishNet** - High-performance and feature-rich alternative to Mirror.
3. **Photon** - Cloud-based networking with scalable multiplayer capabilities.
4. **Unity Netcode** - Unity's official multiplayer framework for small-scale projects.
Setting Up Mirror Networking
Mirror is a popular **high-performance networking solution** for Unity.
1. Install Mirror
To install Mirror, add it via **Unity Package Manager**:
1. Open Unity's Package Manager (Window > Package Manager).
2. Click the **+** button and choose "Add package from Git URL."
3. Enter: `https://github.com/MirrorNetworking/Mirror.git`
4. Click "Add" to install Mirror.
2. Create a Network Manager
Mirror requires a **NetworkManager** component to handle player connections.
using Mirror;
using UnityEngine;
public class MyNetworkManager : NetworkManager
{
public override void OnServerAddPlayer(NetworkConnectionToClient conn)
{
GameObject player = Instantiate(playerPrefab);
NetworkServer.AddPlayerForConnection(conn, player);
}
}
3. Synchronizing Player Movement
Use Mirrorβs **NetworkTransform** to sync player movement.
using Mirror;
using UnityEngine;
public class PlayerMovement : NetworkBehaviour
{
public float speed = 5f;
void Update()
{
if (!isLocalPlayer) return;
float moveX = Input.GetAxis("Horizontal");
float moveY = Input.GetAxis("Vertical");
transform.Translate(new Vector3(moveX, moveY, 0) * speed * Time.deltaTime);
}
}
4. Spawning Players
Players need to spawn in the **networked environment**.
using Mirror;
using UnityEngine;
public class PlayerSpawner : NetworkBehaviour
{
public GameObject playerPrefab;
public override void OnStartServer()
{
GameObject player = Instantiate(playerPrefab, Vector3.zero, Quaternion.identity);
NetworkServer.Spawn(player);
}
}
Building & Testing Multiplayer
To test your multiplayer game:
1. Build your game: **File > Build Settings > PC, Mac, Linux**
2. Open multiple instances of the game.
3. Start one as **Host** and others as **Clients**.
4. Watch players sync across the network!
Conclusion
Multiplayer networking in Unity opens up endless possibilities for **online experiences**. Whether using **Mirror, FishNet, or Photon**, the key to successful networking is **optimizing performance and reducing latency**. Start small, test often, and build your dream multiplayer game!