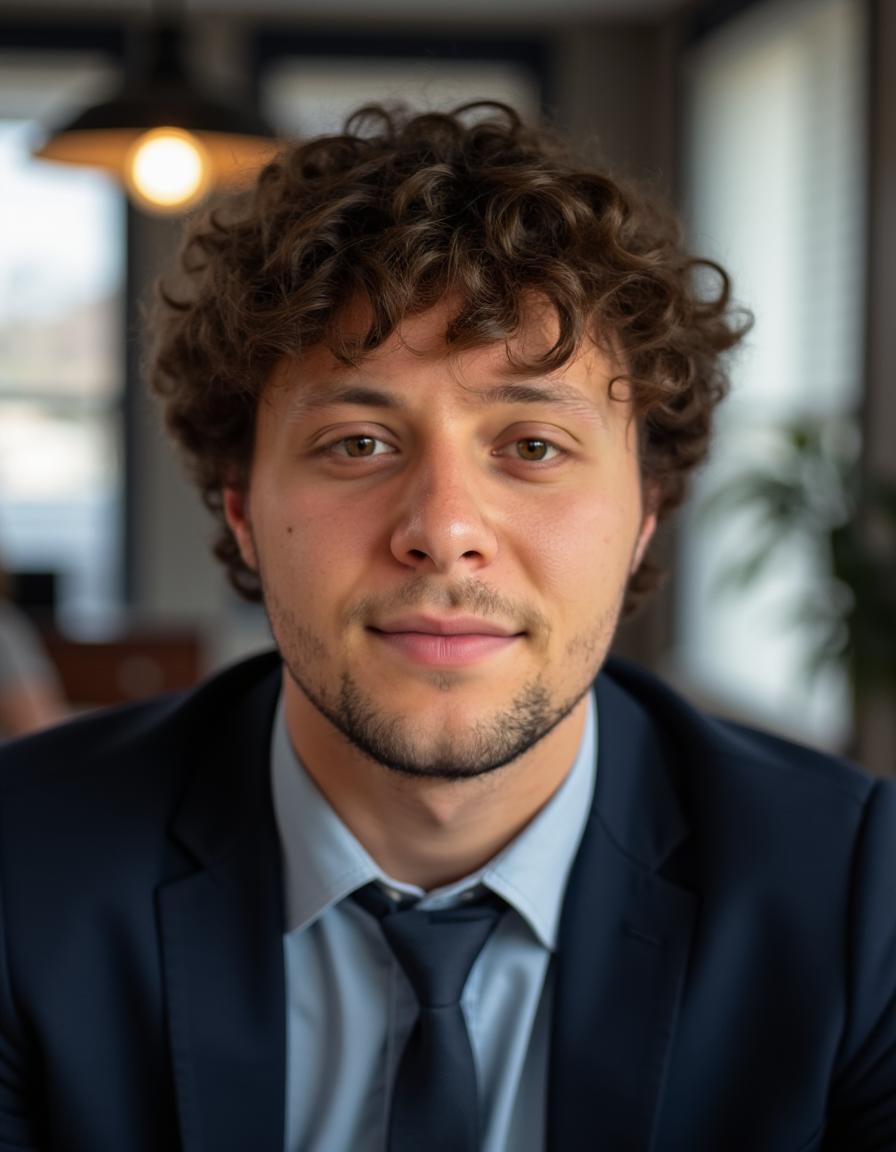
Mastering Laravel: A Comprehensive Guide
Laravel is a robust PHP framework designed to simplify web development. It follows the MVC (Model-View-Controller) architecture, offering clean syntax and built-in tools for authentication, routing, database management, and more.
Why Use Laravel?
Laravel is packed with features that make development efficient and enjoyable:
- Elegant Routing - Define clean and simple routes.
- Blade Templating - Reusable and dynamic views.
- Eloquent ORM - Database interactions made easy.
- Authentication & Authorization - Built-in user management.
- Queue & Jobs - Handle background tasks efficiently.
Getting Started with Laravel
To install Laravel, use Composer:
composer create-project laravel/laravel my-project
Start the local development server:
php artisan serve
Your Laravel app is now running at http://127.0.0.1:8000
.
Creating a New Route
Routes in Laravel are defined inside the routes/web.php
file.
Route::get('/hello', function () {
return "Hello, Laravel!";
});
This route makes http://127.0.0.1:8000/hello
return "Hello, Laravel!"
Working with Controllers
To organize logic, use controllers:
php artisan make:controller HomeController
Now, add a method inside app/Http/Controllers/HomeController.php
:
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class HomeController extends Controller
{
public function index()
{
return view('home');
}
}
Then update your route:
Route::get('/', [HomeController::class, 'index']);
Database Migrations
To create a new database table, use Laravel migrations:
php artisan make:migration create_posts_table --create=posts
Then, run the migration:
php artisan migrate
Conclusion
Laravel streamlines web development, making it faster and more efficient. Keep exploring its rich features!