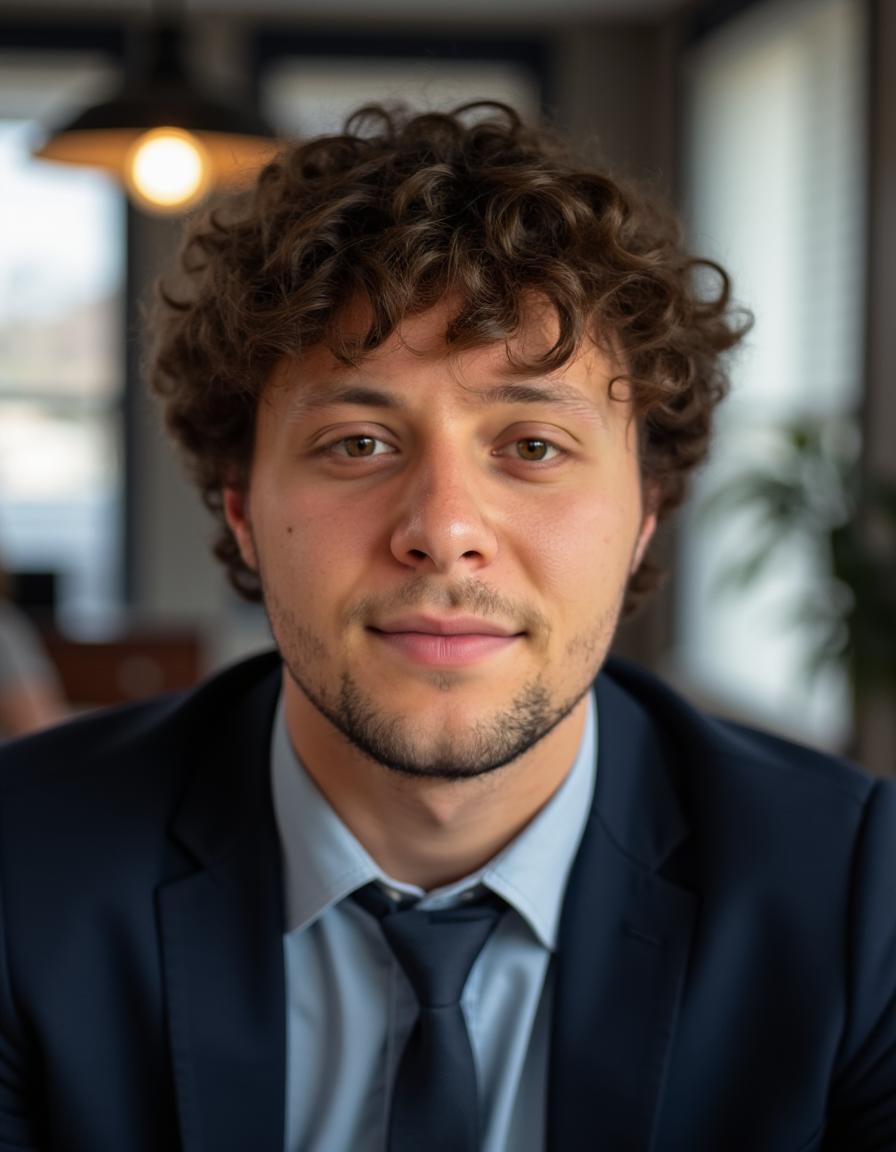
Mastering JavaScript: The Language of the Web
JavaScript is the **core language** of the web, enabling dynamic content, interactivity, and advanced functionalities in modern applications. It runs in the browser, powers APIs, and is a key component of front-end and back-end development.
Why Use JavaScript?
JavaScript is a **versatile** and **powerful** language with features like:
- Client-Side Interactivity – Create **dynamic UI elements**.
- Event-Driven Programming – Respond to **user actions in real time**.
- Asynchronous Processing – Handle **API calls and background tasks**.
- Full-Stack Development – Use **Node.js for backend development**.
Getting Started with JavaScript
To use JavaScript, add a `script` tag to your HTML file:
<script>
console.log("Hello, JavaScript!");
</script>
Declaring Variables
JavaScript uses **let, const, and var** for variable declaration:
let name = "Jayden"; // Can be reassigned
const age = 25; // Cannot be changed
var profession = "Developer"; // Older method, avoid using
Functions in JavaScript
Functions allow you to **reuse code** efficiently:
// Traditional Function
function greet(name) {
return `Hello, ${name}!`;
}
// Arrow Function
const greetArrow = name => `Hello, ${name}!`;
console.log(greet("Jayden"));
console.log(greetArrow("Jayden"));
Event Listeners
JavaScript can respond to user actions with **event listeners**:
document.getElementById("btn").addEventListener("click", function() {
alert("Button clicked!");
});
Asynchronous JavaScript
JavaScript supports **asynchronous operations** using `setTimeout`, `fetch`, and `async/await`:
async function fetchData() {
let response = await fetch("https://jsonplaceholder.typicode.com/posts/1");
let data = await response.json();
console.log(data);
}
fetchData();
JavaScript vs. Other Languages
- 🌎 Runs in Browsers – No setup needed.
- ⚡ Fast Execution – Optimized engines.
- 🔗 Full-Stack Capable – Works with Node.js.
- 🎯 Event-Driven – Ideal for UI interactions.
Conclusion
JavaScript is **the most widely used language on the web**. Whether you're building simple animations or complex applications, mastering JavaScript is essential for modern development.