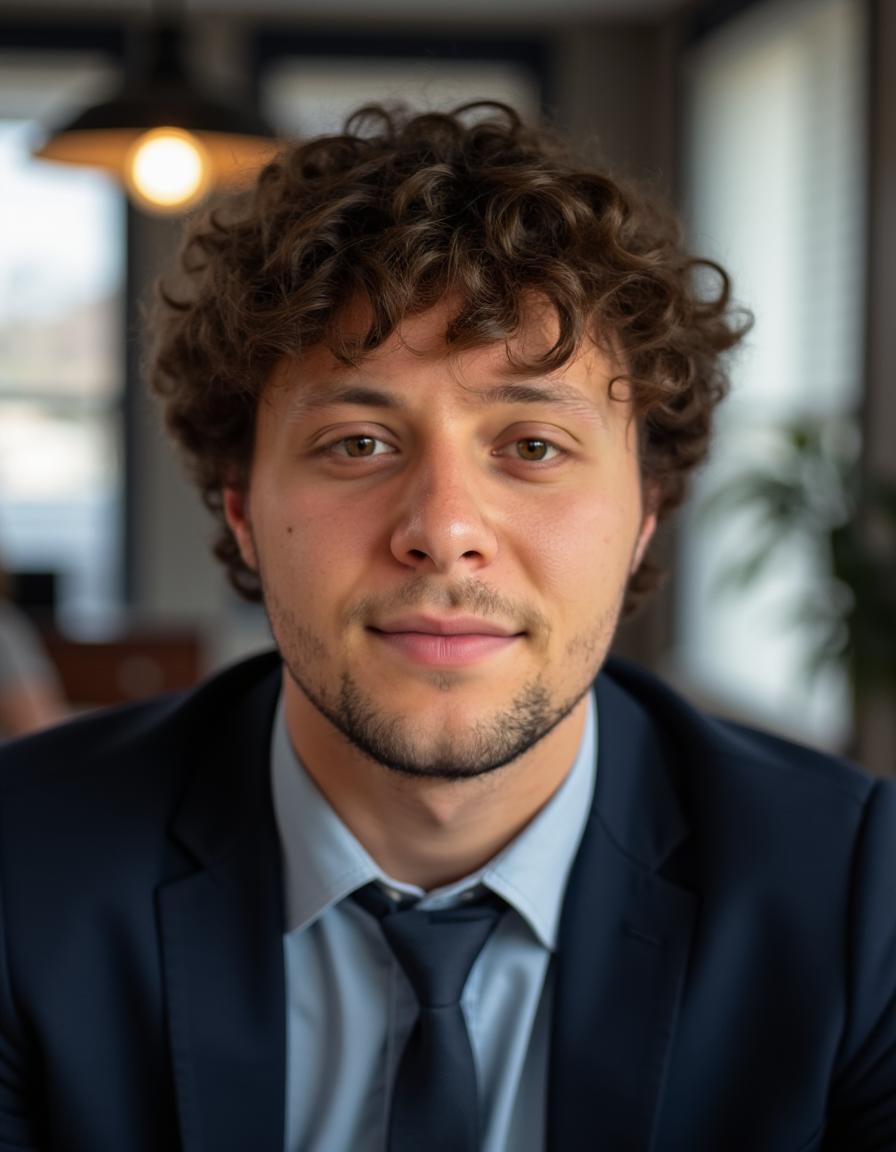
Mastering GraphQL: Efficient API Development
GraphQL is a **modern API query language** that allows clients to request **only the data they need**. Unlike REST APIs, which require multiple endpoints for different resources, GraphQL enables **flexible and efficient data fetching** from a single endpoint.
Why Use GraphQL?
GraphQL offers **powerful advantages** over traditional REST APIs:
- Single Endpoint – Fetch all required data in **one request**.
- Strongly Typed Schema – **Self-documenting API** with clear data structures.
- Efficient Queries – Fetch **only what you need**, reducing over-fetching.
- Better Developer Experience – **Introspective API** allows easy discovery.
Getting Started with GraphQL
To install GraphQL for a Node.js backend, use:
npm install express express-graphql graphql
Defining a GraphQL Schema
GraphQL requires a schema that defines **types and queries**:
const { GraphQLObjectType, GraphQLSchema, GraphQLString } = require("graphql");
const RootQuery = new GraphQLObjectType({
name: "RootQueryType",
fields: {
message: {
type: GraphQLString,
resolve() {
return "Hello, GraphQL!";
}
}
}
});
module.exports = new GraphQLSchema({ query: RootQuery });
Setting Up a GraphQL API
Now, set up an **Express server** to handle GraphQL requests:
const express = require("express");
const { graphqlHTTP } = require("express-graphql");
const schema = require("./schema");
const app = express();
app.use("/graphql", graphqlHTTP({
schema,
graphiql: true
}));
app.listen(4000, () => console.log("Server running on port 4000"));
Now, visit http://localhost:4000/graphql
to start querying!
Running a GraphQL Query
Once your API is running, you can make **queries like this:**
{
message
}
This will return:
{ "message": "Hello, GraphQL!" }
GraphQL vs. REST
GraphQL improves API development in several key areas:
- 📡 Single Endpoint – No more multiple REST routes.
- 🎯 Fetch Only What You Need – Prevents over-fetching.
- ⚡ Fast Queries – Optimized for speed.
- 📜 Strong Typing – Schema ensures consistency.
Conclusion
GraphQL is a **game-changer for API development**, providing **efficiency, flexibility, and scalability**. Whether you're building a frontend-heavy app or a complex microservices architecture, GraphQL streamlines data fetching and management.