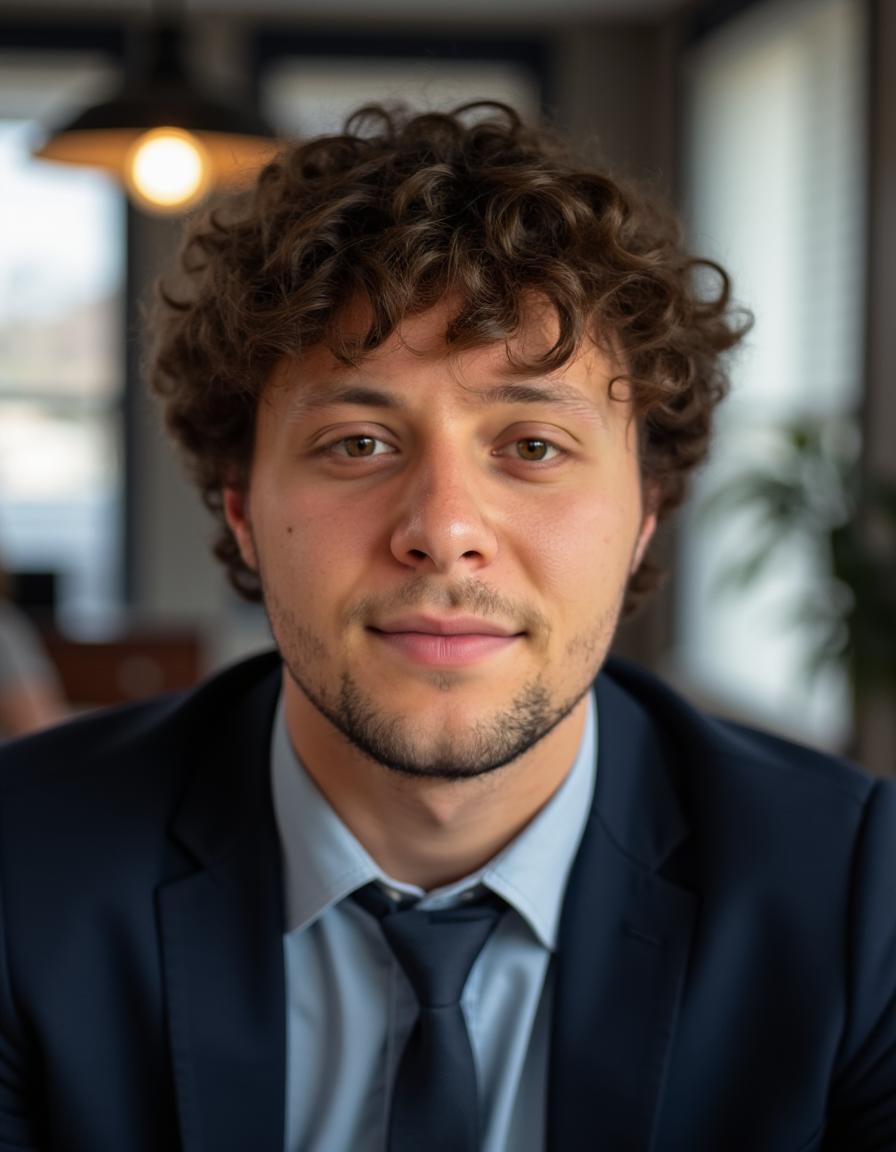
Game UI/UX: Designing Immersive Player Experiences
Game UI/UX (User Interface and User Experience) plays a crucial role in game design, ensuring that players can navigate, interact, and enjoy the game seamlessly. A well-designed UI/UX enhances immersion, engagement, and overall satisfaction.
Why is Game UI/UX Important?
Game UI/UX is essential for:
- Clarity & Accessibility - Information must be presented clearly and intuitively.
- Immersion - UI should complement the gameβs aesthetics and not distract from gameplay.
- Navigation - Menus, HUD elements, and controls must be user-friendly.
- Feedback - UI should provide instant responses to player actions.
Key Components of Game UI
1. Heads-Up Display (HUD)
The HUD displays essential game information, such as health, score, and abilities.
using UnityEngine;
using UnityEngine.UI;
public class HUDManager : MonoBehaviour
{
public Text healthText;
public Slider healthBar;
void UpdateHUD(int health, int maxHealth)
{
healthText.text = "Health: " + health + "/" + maxHealth;
healthBar.value = (float)health / maxHealth;
}
}
2. Menus & Navigation
Menus allow players to interact with the game settings, inventory, and more.
using UnityEngine;
using UnityEngine.SceneManagement;
public class MainMenu : MonoBehaviour
{
public void StartGame()
{
SceneManager.LoadScene("GameScene");
}
public void QuitGame()
{
Application.Quit();
}
}
3. Inventory & Equipment UI
Players need an intuitive inventory system to manage items and gear.
using UnityEngine;
using UnityEngine.UI;
using System.Collections.Generic;
public class InventoryUI : MonoBehaviour
{
public List inventorySlots;
public Sprite emptySlotSprite;
public void UpdateInventoryUI(List itemIcons)
{
for (int i = 0; i < inventorySlots.Count; i++)
{
inventorySlots[i].sprite = i < itemIcons.Count ? itemIcons[i] : emptySlotSprite;
}
}
}
4. Dialogue & Storytelling UI
Dialogue UI enhances player engagement with characters and storytelling.
using UnityEngine;
using UnityEngine.UI;
public class DialogueSystem : MonoBehaviour
{
public Text dialogueText;
public GameObject dialogueBox;
public void ShowDialogue(string text)
{
dialogueBox.SetActive(true);
dialogueText.text = text;
}
public void HideDialogue()
{
dialogueBox.SetActive(false);
}
}
5. Player Feedback UI
Animations, visual effects, and audio cues are crucial for responsive UI.
using UnityEngine;
using UnityEngine.UI;
public class UIFeedback : MonoBehaviour
{
public Image damageEffect;
public void ShowDamageEffect()
{
damageEffect.color = new Color(1, 0, 0, 0.5f);
Invoke("HideDamageEffect", 0.3f);
}
void HideDamageEffect()
{
damageEffect.color = new Color(1, 0, 0, 0);
}
}
Conclusion
A well-designed Game UI/UX enhances **immersion, usability, and player experience**. Whether itβs HUDs, inventory, or responsive feedback, designing with the player in mind ensures a seamless gaming experience.