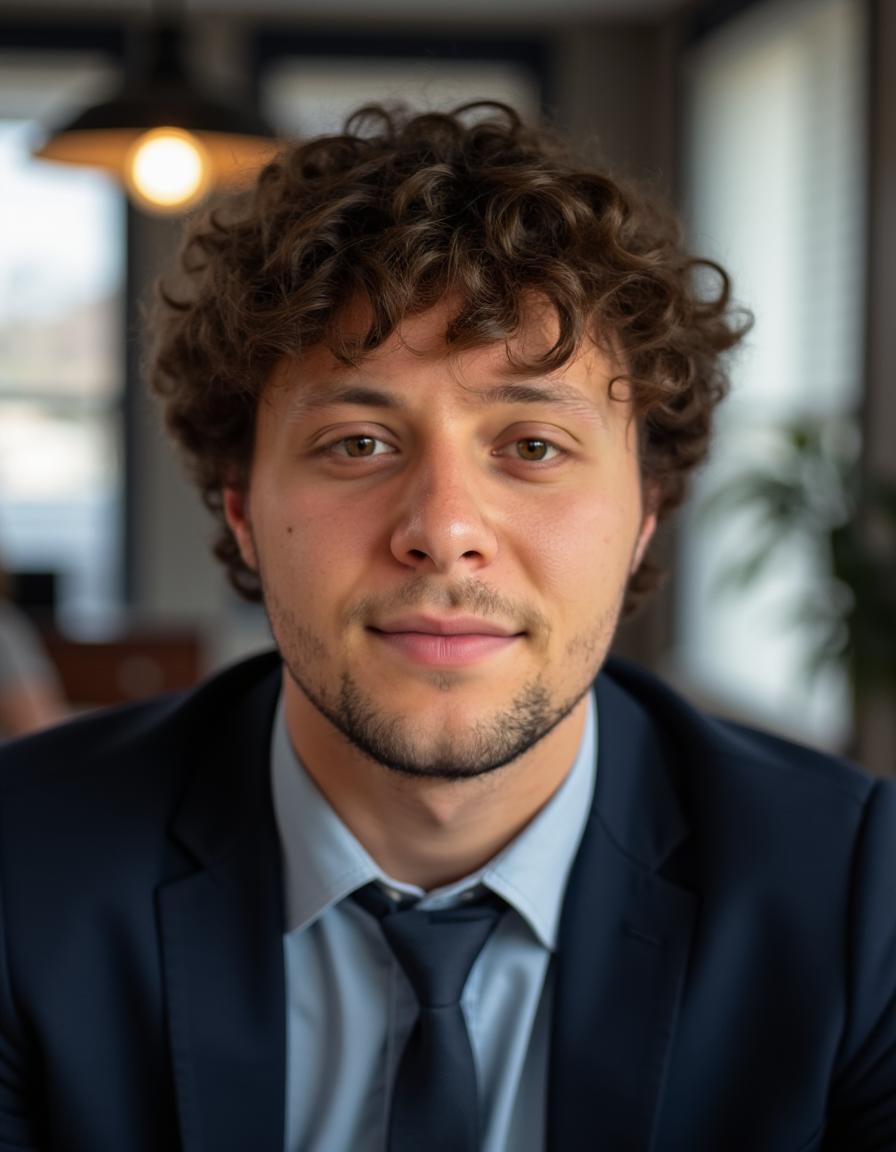
Animation & VFX: Bringing Games to Life
Animation and Visual Effects (VFX) are crucial in game development, enhancing immersion and making interactions feel more dynamic and impactful. From character animations to environmental effects, mastering these elements is key to creating a visually stunning game.
Why Are Animation & VFX Important?
Animation & VFX improve the player's experience by:
- Creating Realistic Movements - Smooth animations make characters and objects feel natural.
- Enhancing Feedback - Visual cues help players understand actions and interactions.
- Improving Immersion - Special effects like lighting, particles, and shaders add depth.
- Making Combat & Actions More Satisfying - Well-designed effects amplify impact.
Character Animation in Unity
Unity uses the Animator system to handle character animations.
using UnityEngine;
public class CharacterAnimation : MonoBehaviour
{
private Animator animator;
void Start()
{
animator = GetComponent();
}
void Update()
{
if (Input.GetKey(KeyCode.W))
{
animator.SetBool("isRunning", true);
}
else
{
animator.SetBool("isRunning", false);
}
}
}
Creating Particle Effects
Unityβs Particle System allows for dynamic VFX like explosions, magic spells, and fire.
using UnityEngine;
public class ParticleEffect : MonoBehaviour
{
public ParticleSystem explosionEffect;
void Update()
{
if (Input.GetKeyDown(KeyCode.Space))
{
Instantiate(explosionEffect, transform.position, Quaternion.identity);
}
}
}
Shader Effects for Stunning Visuals
Shaders allow for dynamic, real-time visual effects like glow, distortion, and reflections.
Shader "Custom/GlowEffect"
{
Properties {
_Color ("Main Color", Color) = (1,1,1,1)
_Glow ("Glow Intensity", Range(0,1)) = 0.5
}
SubShader {
Tags { "RenderType"="Opaque" }
Pass {
CGPROGRAM
#pragma vertex vert
#pragma fragment frag
fixed4 _Color;
float _Glow;
struct appdata {
float4 vertex : POSITION;
};
struct v2f {
float4 pos : SV_POSITION;
};
v2f vert (appdata v) {
v2f o;
o.pos = UnityObjectToClipPos(v.vertex);
return o;
}
fixed4 frag (v2f i) : SV_Target {
return _Color * _Glow;
}
ENDCG
}
}
}
Screen Shake for Impactful Moments
Adding a subtle screen shake effect enhances explosions, attacks, and movement.
using UnityEngine;
public class ScreenShake : MonoBehaviour
{
public Transform cameraTransform;
public float shakeDuration = 0.2f;
public float shakeIntensity = 0.3f;
private Vector3 originalPosition;
void Start()
{
originalPosition = cameraTransform.localPosition;
}
public void TriggerShake()
{
StartCoroutine(Shake());
}
private IEnumerator Shake()
{
float elapsed = 0.0f;
while (elapsed < shakeDuration)
{
float x = Random.Range(-shakeIntensity, shakeIntensity);
float y = Random.Range(-shakeIntensity, shakeIntensity);
cameraTransform.localPosition = new Vector3(x, y, originalPosition.z);
elapsed += Time.deltaTime;
yield return null;
}
cameraTransform.localPosition = originalPosition;
}
}
Conclusion
Animation & VFX are essential for making games engaging and visually impressive. Mastering animations, particle effects, shaders, and camera feedback will significantly enhance your game's quality.